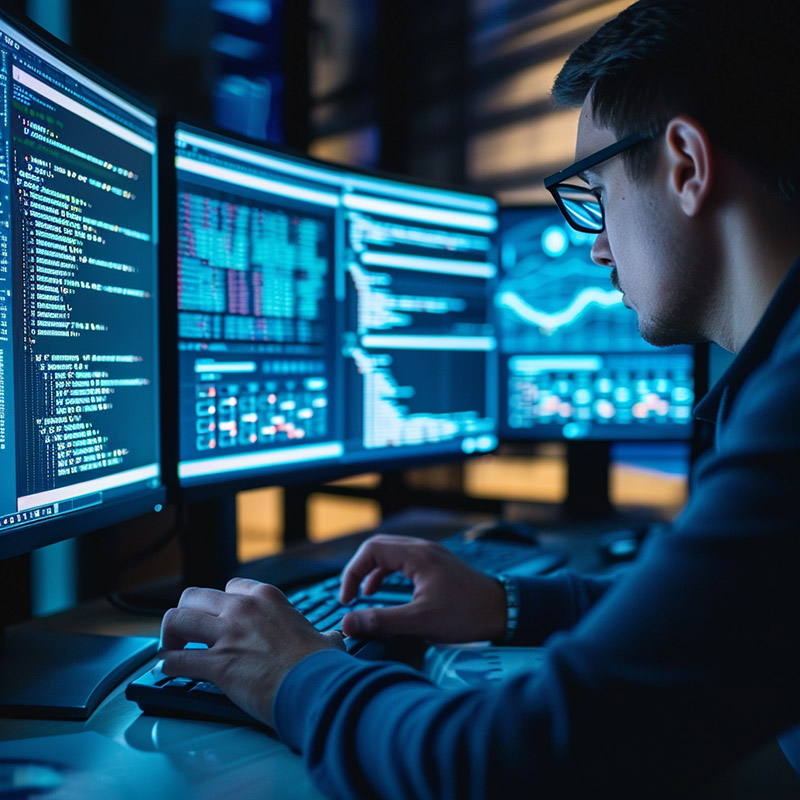
Basics of UNIX Shell Programming
1. Understanding the Shell
The shell is a command-line interface for interacting with the operating system. Common shells include Bash, Korn shell, and C shell.
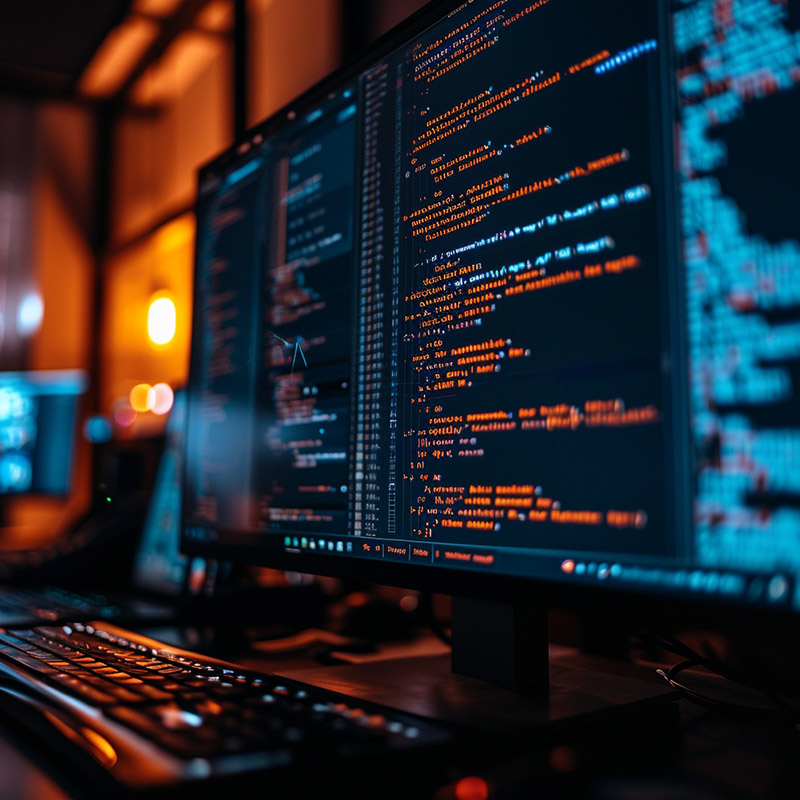
2. Basic Commands
- ls: Lists directory contents.
- cd: Changes the current directory.
- pwd: Displays the current directory path.
- cp: Copies files and directories.
- mv: Moves or renames files and directories.
- rm: Removes files and directories.
- echo: Displays a line of text.
- cat: Concatenates and displays file contents.
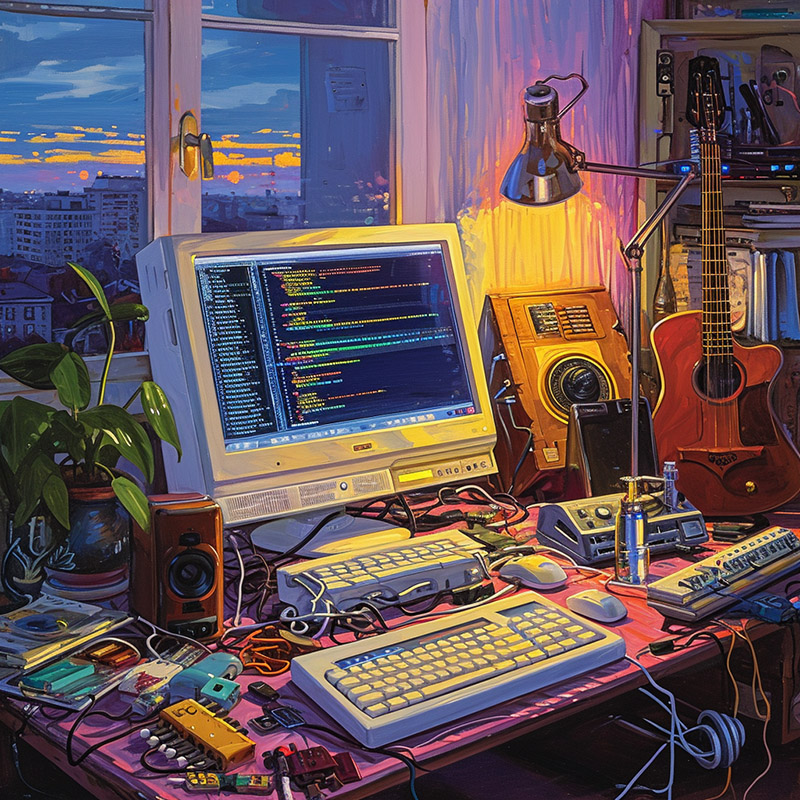
3. File Permissions and Ownership
Understanding and modifying file permissions and ownership using chmod and chown.
4. Redirection and Piping
- Redirection using >, >>, <.
- Pipes (|) to send the output of one command as the input to another.
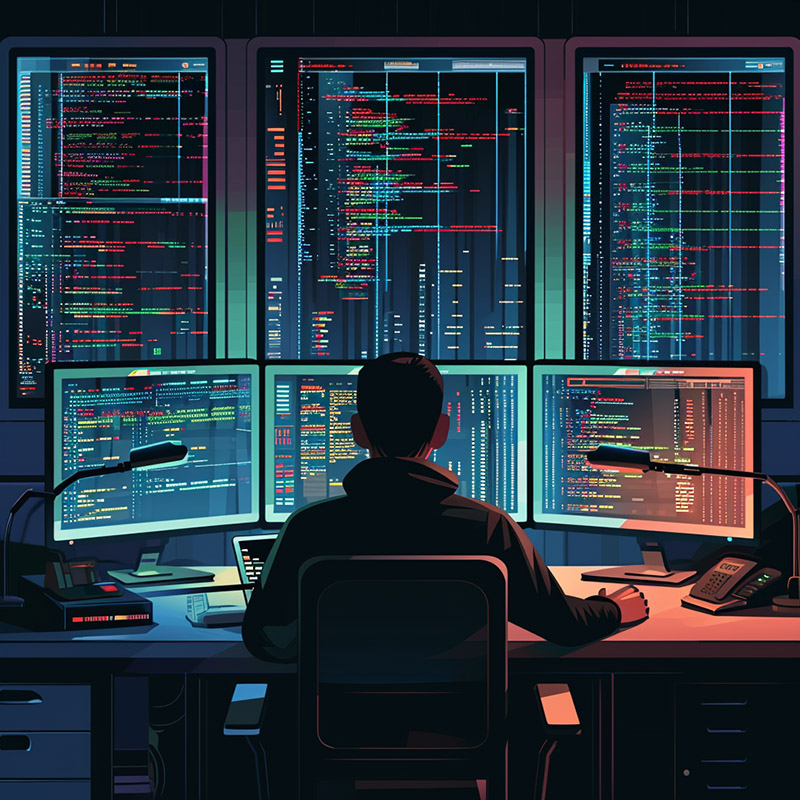
5. Environment Variables
Variables used by the operating system and applications, like PATH, HOME, and USER.
6. Shell Scripting
- Writing and executing scripts with a .sh extension.
- Using variables, arguments, and input in scripts.
- Control structures such as loops and conditionals.
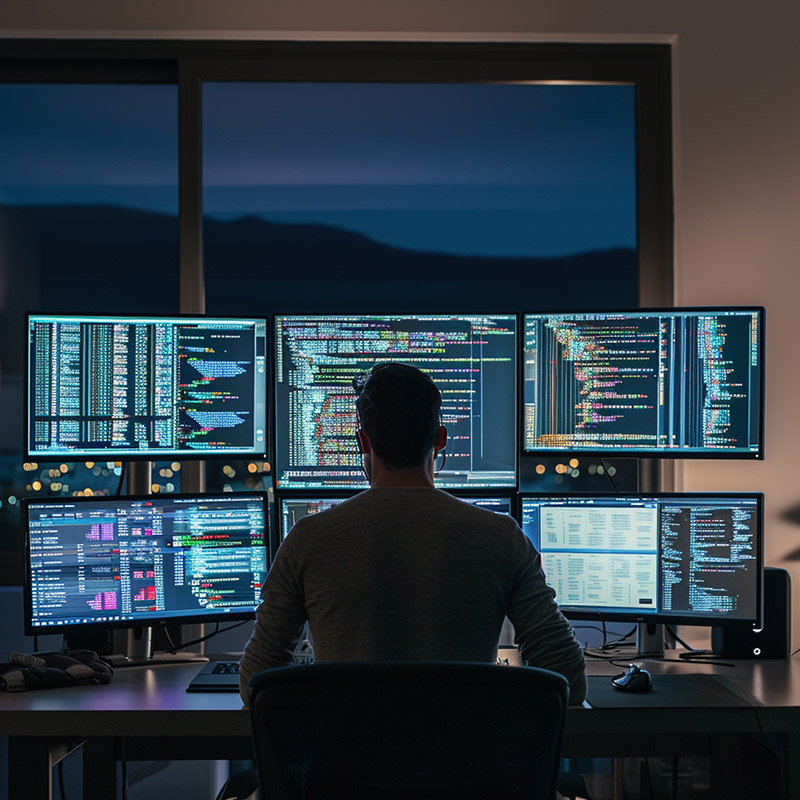
7. Text Processing Tools
- grep: For searching within files.
- sed: A stream editor for modifying files.
- awk: A programming language for text processing.
8. Process Management
- Viewing and killing processes.
- Managing background and foreground processes.
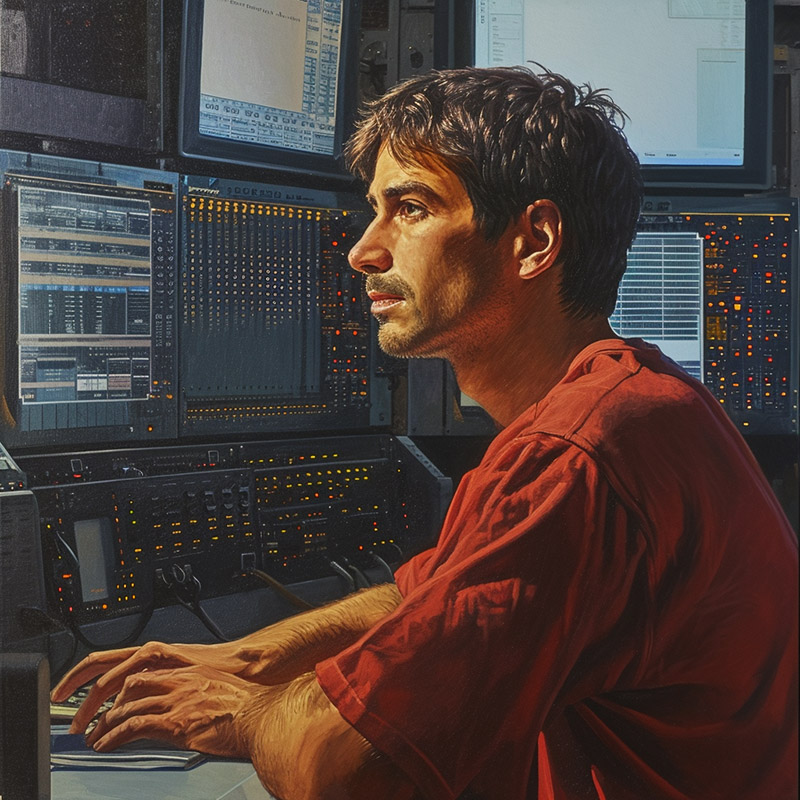
9. Networking Commands
Basic networking commands like ping, ssh, and scp.
10. Advanced Scripting
Complex scripting techniques including functions, advanced variable types, and regular expressions.
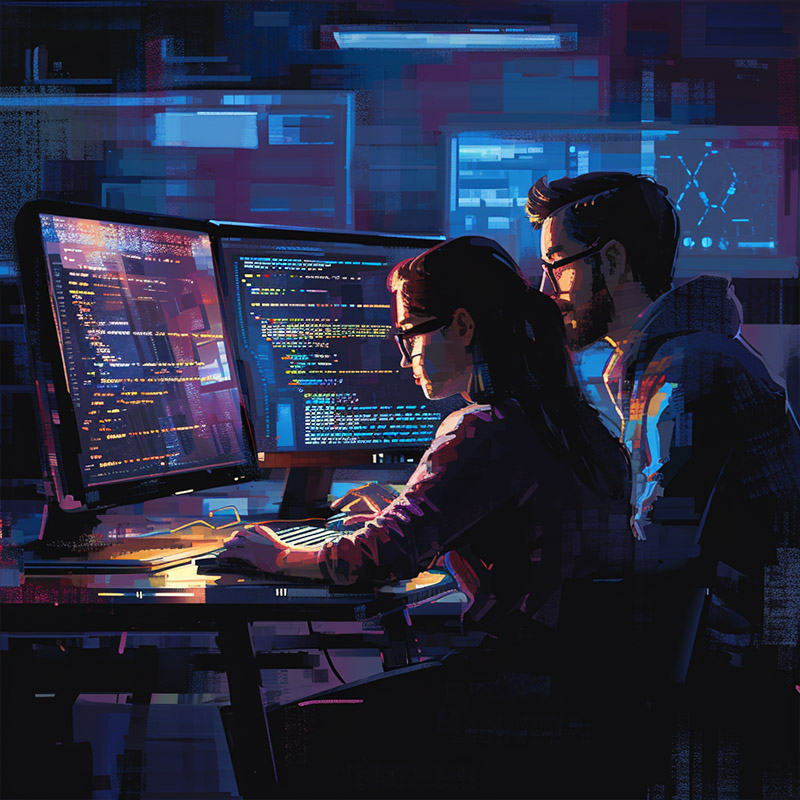
Unix Shell Programming Glossary
Shell: A command-line interpreter that provides a user interface for the Unix operating system.
Bash: A widely-used Unix shell and command language, standing for Bourne Again SHell.
Script: A text file containing a series of commands that can be executed by a shell.
Command: An instruction or utility provided by the shell to perform specific tasks.
Argument: A string of text or data provided to a command to modify its behavior or output.
Pipe: A method for passing the output of one command directly into another command.
Environment Variable: A dynamic value stored within the shell that can influence the behavior of running processes.
Home Directory: The default working directory for a user when they log into the Unix system.
Root: The top-level directory in a Unix filesystem, or the superuser account with full privileges.
Permission: Access rights granted to users and groups to read, write, or execute files or directories.
Path: A file or directory's unique location in the filesystem.
Wildcard: Characters like '*' or '?' used to match patterns in filenames or commands.
Standard Input (stdin): A stream through which data is sent to commands or programs.
Standard Output (stdout): A stream where data output from commands or programs is sent.
Standard Error (stderr): A stream used for outputting error messages from commands or programs.
Process: An instance of a running program.
PID (Process ID): A unique number assigned to each process running in Unix.
Fork: Creating a copy of a process.
Signal: A notification sent to a process to request it to stop, pause, or terminate.
Alias: A shortcut to reference a command or series of commands.
Variable: A named storage location that holds a value which can change during script execution.
Function: A block of code that can be reused in a shell script.
Loop: A sequence of instructions that repeats until a certain condition is reached.
Conditional Statement: A statement that executes commands based on specified conditions.
Exit Status: A numerical value returned by a command to indicate its execution status.
Grep: A command used to search text using pattern matching.
Sed: A stream editor used to perform basic text transformations.
Awk: A programming language used for text processing and manipulation.
Redirection: A method to change the standard input/output channels of a command.
Chmod: A command to change file access permissions.
Cron: A Unix utility for scheduling tasks or commands at specific intervals.